Using the model builder interface to combine columns should add to the efficiency of SQL queries. When using inheritance, we usually define shared properties on base types, but there might be an instance where that’s not the case. Let’s look at an example where two models in our hierarchy share the same property name without inheriting from the base type. While it may feel like this would be a “cleaner” approach to data modeling, it comes at an expensive cost when querying across the hierarchy.
It’s a convenience that has saved every C# developer countless hours of shuffling index values in format strings. EF Core allows for the use of string interpolation when using raw SQL. Still, developers must be careful not to refactor their code into an SQL injection vulnerable state inadvertently. Let’s start with a parameterized SQL using an interpolated string.
Welcome To Learn Entity Framework Core
Entity Framework will take care of creating the connection to your database, creating the objects you can use to manipulate the database, and give you methods to work with what is entity framework your data. Perhaps, you have an idea for your application that would benefit from having a database. You will be able to perform CRUD operations via your application.
- It’s worth experimenting with AsSplitQuery, as it can speed up queries and reduce network traffic by removing data duplication.
- While we can install the tooling globally, it’s best to version the CLI tooling and EF Core together in your solution.
- The .NET Framework provides us with three different types of data providers – ADO.NET, OLEDB, and ODBC.
- It enables the developers to work with the data using the objects of domain-specific classes without focussing on the database tables and columns where the data is stored.
- It uses the model to create the database and build the tables and columns of the tables.
The output parameter must be declared explicitly in .NET and map to an output parameter in the function import definition. After adding a property, the domain model and the database are out of sync, and it is required to match the database with the model. When you make changes to the domain class or when you add new classes, a new migration must be defined in the Migrations folder. A typical example of this kind is adding a new property to a domain class.
The Ultimate Guide to Normalization in SQL
There are two different ways to approach the use of Entity Framework in your application. These different approaches have their own benefits and it’s up to you to decide which one works best for your needs. Both ORMs are great and work with any kind of project, from small-scale to massive enterprise-level applications. Having .NET Framework, Visual Studio, and SQL Server installed on your computer is good. ORMs Tools are used to increase the developer’s productivity by reducing the redundant task of doing CRUD operations against a database in a .NET Application.
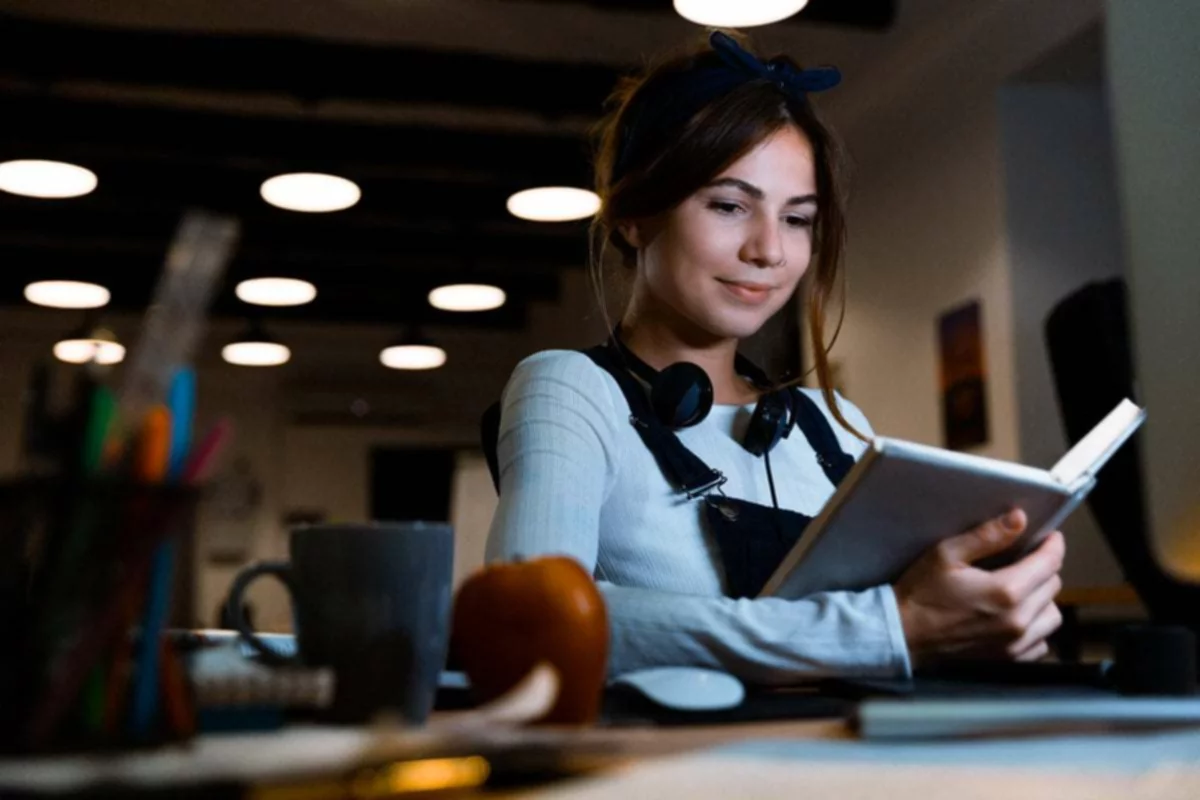
Keep in mind nothing will really happen in the DB if you don’t call SaveChanges. You need to name the entity and the table the same way, except, there are some conventions that EF follows and you may be able to override some of those. Notice that using the attribute to indicate the type of action, I can name the method whatever I want. When you are dealing with the database, we have what we call CRUD operations.
Finally, when the data is retrieved, we have to create objects of Employee and Department to populate them with recovered data. The above entire thing is tedious, but through Entity Framework, it is easy; those things can be done automatically; we need to provide DB Schema to EF. Let’s see the following steps to create an application using EF.